Restricted door
Restricted doors, also commonly called VIP doors, are used to restrict access to a certain area to only certain users. There are many restrictions that one could want to apply to a restricted door, and this article can not cover them all. It will however cover the most common restrictions:
- Being a certain player.
- Being the creator of the place.
However, you should always think before making a restricted door that restricts access to a certain area. In most cases, there will be a better way to do it. The problem is that restricted doors are often overused. Sometimes, they are used to protect a room that contains certain items. Yet, these items could be given automatically to the players, instead of them having to enter the room every time they die. Try to avoid using a restricted door if you can do it in a cleaner way.
Building the door
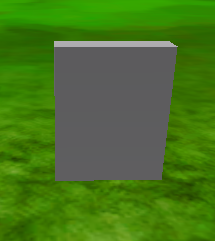
Building the door should not be a problem, but I am still going to describe it. First, you will need to create the door. Usually, you will want it to be of the size of a character, so players can get through. You will probably want the door to be anchored too, unless you expect it to move whenever a player touches it...
The size of a player's character is 4 x 5 x 1, so you will probably want your door to have that size. However, you can't get exactly that size with the Brick FormFactor. Therefore, you will probably want to change the FormFactor to Symmetric, so you can get the exact size. Another solution would be to simply make the door slightly bigger than the exact size of a character. Usually, you will also want to build a room around the door, but that is out of the scope of this tutorial.
Scripting the door
Structure
First, we will need to create a script in the door. Go in the "Insert" menu and choose "Object...". Then, use the dialog that appears to insert a script in the door. Once you have a script in your door, open it to edit it in the script editor.
Because the door will be manipulated a lot in the script, it would be a good idea to define a variable that contains a reference to it. I'll call that variable door, but you can call it whatever you want.
local door = script.Parent
You will probably want to be able to open and close the door. Let's make a function to open the door and another to close it.
There are many ways to open a door, but, for this tutorial, we will choose one that makes the door progressively disappear, as you can see here:
(don't worry, your door will fade faster than that. The animation here is slower than how fast your door will actually fade)
We will use a loop to make the door fade, and we will use its Transparency property to change its opacity. Then, finally, we will change the door's CanCollide property to allow players to walk through it.
In case you weren't sure how to write the code, here is an example that works. However, you are encouraged to write your own code, as that is how you will learn.
local door = script.Parent
local function open()
-- This function will open the door.
door.CanCollide = false -- Make players able to walk through the door.
for transparency = 0, 1, .1 do
door.Transparency = transparency
wait(.1)
end
end
If there is an open function, there is of course also a close function. That close function does exactly the opposite of what the open function does, so you should be able to write the code yourself. If not, here is the code as I wrote it, but remember you are encouraged to write it yourself.
local door = script.Parent
local function open()
-- This function will open the door.
door.CanCollide = false -- Make players able to walk through the door.
for transparency = 0, 1, .1 do
door.Transparency = transparency
wait(.1)
end
end
local function close()
-- This function will close the door.
for transparency = 1, 0, -.1 do
door.Transparency = transparency
wait(0.1)
end
door.CanCollide = true -- Make players unable to walk through the door.
end
You probably want the door to open when it is touched, and, therefore, it should use the Touched event. Therefore, let's connect the Touched event to a function. Then, we will check if the part that touched the door is in a character. Here is the code that I wrote.
local door = script.Parent
local function open()
-- This function will open the door.
door.CanCollide = false -- Make players able to walk through the door.
for transparency = 0, 1, .1 do
door.Transparency = transparency
wait(.1)
end
end
local function close()
-- This function will close the door.
for transparency = 1, 0, -.1 do
door.Transparency = transparency
wait(.1)
end
door.CanCollide = true -- Make players unable to walk through the door.
end
-- This function returns the player a certain part belongs to.
local function get_player(part)
-- iterate over each player
for _, player in ipairs(game.Players:GetPlayers()) do
-- if the part is within the player's character
if part:IsDescendantOf(player.Character) then
-- return the player
return player
end
end
end
door.Touched:connect(function(part)
-- If it isn't a character that touched the door, then we ignore it.
local player = get_player(part)
if not player then return end
end)
Now, what do we want to do when the door is touched? We want to check if the player corresponds to one of the restrictions. That brings me to the next section...
Restrictions
There are many ways to check if the player corresponds to one of the restrictions, but we will use a simple system in this case. However, you are encouraged to try other ways to do it, as that will help you to learn.
The system we will use in this example is simple. We will separate all the restrictions by the or keyword. All the restrictions will be under the form of Boolean expressions. If any of these Boolean expressions is true, then the door will open.
If any of the restrictions evaluates to true, it will open the door, wait 4 seconds and then close it. You can see the code I wrote below.
local door = script.Parent
local function open()
-- This function will open the door.
door.CanCollide = false -- Make players able to walk through the door.
for transparency = 0, 1, .1 do
door.Transparency = transparency
wait(.1)
end
end
local function close()
-- This function will close the door.
for transparency = 1, 0, -.1 do
door.Transparency = transparency
wait(.1)
end
door.CanCollide = true -- Make players unable to walk through the door.
end
-- This function returns the player a certain part belongs to.
local function get_player(part)
-- iterate over each player
for _, player in ipairs(game.Players:GetPlayers()) do
-- if the part is within the player's character
if part:IsDescendantOf(player.Character) then
-- return the player
return player
end
end
end
door.Touched:connect(function(part)
-- If it isn't a character that touched the door, then we ignore it.
local player = get_player(part)
if not player then return end
local allow = (
false
)
if allow then
open()
delay(4, close)
end
end)
Now, we need to put some restrictions in there. A restriction, in this case, is
Let's see some common restrictions you might want to apply.
Being a certain player
Often, you will want to authorize certain specific players to go through the door. In a such case, this is probably what you would do.
You have probably already guessed how to apply this restriction, but, if you didn't, it is simple. You just check if the player's name is equal to a certain string:
player.Name == "PlayerName"
Being the creator of the place
This restriction could be achieved by simply comparing the player's name with a certain predefined name (the name of the place's owner) and checking if they are equal. However, this way of doing it is useful, as it will work correctly in any place. For instance, you don't need to edit the script before giving it to a friend, as it will adapt to the place. It uses the CreatorId property of the DataModel and compares it to the player's id. If they are equal, then the player is the owner. Therefore, it works in any place and you don't even need to change your name to the name of your friend when giving it to him.
Here is how you apply this restriction:
game.CreatorId == player.userId
Using restrictions
Using restrictions is simple: you just separate them by 'or'. Let's refresh our memory by looking back at where we were in the code:
local door = script.Parent
local function open()
-- This function will open the door.
door.CanCollide = false -- Make players able to walk through the door.
for transparency = 0, 1, .1 do
door.Transparency = transparency
wait(.1)
end
end
local function close()
-- This function will close the door.
for transparency = 1, 0, -.1 do
door.Transparency = transparency
wait(.1)
end
door.CanCollide = true -- Make players unable to walk through the door.
end
-- This function returns the player a certain part belongs to.
local function get_player(part)
-- iterate over each player
for _, player in ipairs(game.Players:GetPlayers()) do
-- if the part is within the player's character
if part:IsDescendantOf(player.Character) then
-- return the player
return player
end
end
end
door.Touched:connect(function(part)
-- If it isn't a character that touched the door, then we ignore it.
local player = get_player(part)
if not player then return end
local allow = (
false
)
if allow then
open()
delay(4, close)
end
end)
Now, let's add our restrictions in there! Here is an example that will allow the following players through:
- You, the creator.
- Some predefined players.
Here is the example:
local door = script.Parent
local function open()
-- This function will open the door.
door.CanCollide = false -- Make players able to walk through the door.
for transparency = 0, 1, .1 do
door.Transparency = transparency
wait(.1)
end
end
local function close()
-- This function will close the door.
for transparency = 1, 0, -.1 do
door.Transparency = transparency
wait(.1)
end
door.CanCollide = true -- Make players unable to walk through the door.
end
-- This function returns the player a certain part belongs to.
local function get_player(part)
-- iterate over each player
for _, player in ipairs(game.Players:GetPlayers()) do
-- if the part is within the player's character
if part:IsDescendantOf(player.Character) then
-- return the player
return player
end
end
end
door.Touched:connect(function(part)
-- If it isn't a character that touched the door, then we ignore it.
local player = get_player(part)
if not player then return end
local allow = (
player.Name == "MrDoomBringer" or
player.Name == "JulienDethurens" or
player.Name == "blocco" or
player.Name == "NXTBoy" or
game.CreatorId == player.userId
)
if allow then
open()
delay(4, close)
end
end)
Going further
The door already works, but let's go further. Let's see what we could do to go further than that and make the door even better.
Making the door kill
One thing you probably already saw in some places is a restricted door that kills players if they're not allowed through. In some cases, you might want to use a such mechanism, for example, because of the effect it provides, or because it makes the door more secure (if a player isn't allowed through, he can still go through if a player that is allowed through opens the door for him). If we wanted to make the door kill players that are not allowed through, we could use the else statement to kill the player if the value of the allow variable is false. However, the code will not be provided here, you can write it yourself.
Terminology
This is a list of some of the terms that were used in this article, as well as their definition, in the context of the article.
- restriction
- A Boolean expression that evaluates to true or false depending on the value of the player variable.
- identifier
- An unique integer representing a specific thing out of many things of the same kind. Often abbreviated to "id".
- terminology
- The vocabulary of technical terms used in a particular field, in this case, the current article.