Circle
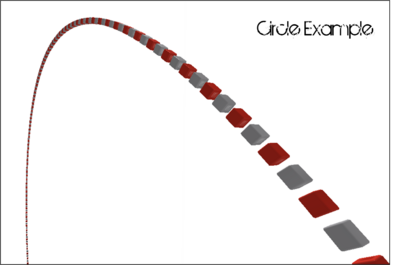
Goal
This is not a Part with the shape of a Ball. This is instead a rather hollow outline pixelated with many small bricks. In fact, you won't be touching bricks at all -- you will only be scripting.
Steps
- Create a new, blank, map in Roblox Studio.
- Insert > Object > Script
- Insert the following into that script (the explanation is below):
for angle = 1, 360 do
local p = Instance.new('Part', Workspace)
p.Size = Vector3.new(1, 1, 1)
p.Anchored = true
p.CFrame = CFrame.new(0, 120, 0) --Start at the center of the circle
* CFrame.Angles(math.rad(angle), 0, 0) --Rotate the brick
* CFrame.new(0, 0, 100) --Move it out by 100 units
wait()
end
Explanation
A circle has 360 degrees. You are going to create 360 little bricks for your circle. The "for" loop runs 360 times. The "math.rad(angle )" converts the angle, which is in degrees, into Radians.
Each time the "for" loop runs, it:
- Creates a new 'part', of which the parent is "game.Workspace".
- The part's name is "Brick"
- The Brick's size is (1, 1, 1) -- remember, we wanted little bricks.
- All the bricks are anchored, so that they don't fall down.
Now comes the tricky bit. Here we use CFrame composition, to avoid using trigonometry. We start off with a CFrame at the center of our circle. Then we rotate by the angle we need. Finally, we move the brick out from the center of the circle. Because this is applied after the rotation, the brick moves relative to the new orientation.
Run this, and you should get a nice little circle.
Advanced
The above example is a rather large circle. Let's say you want a smaller one. You'll have to do two things:
- Reduce the distance you move out from the center of the circle
- Reduce the number of iterations (which reduces the number of bricks).
for angle = 1, 360, 3 do
local p = Instance.new('Part')
p.Parent = Workspace
p.Size = Vector3.new(1,1,1)
p.Anchored = true
p.CFrame = CFrame.new(0, 75, 0) --Start at the center of the circle
* CFrame.Angles(math.rad(angle), 0, 0) --Rotate the brick
* CFrame.new(0, 0, 50) --Move it out by 50 units
wait()
end
Ellipses
Ellipses are quite similar geometrically to circles. You just need to make one dimension (x or y) longer than the other:
for i = 1, 360, 2 do
local theta = math.rad(i)
local p = Instance.new('Part')
p.Parent = Workspace
p.Size = Vector3.new(1, 1, 1)
p.Anchored = true
p.Position = Vector3.new(100 * math.cos(theta), 0, 50 * math.sin(theta))
wait()
end
Notice here that the x dimension is 100*math.cos(i), and the y dimension is now only 50*math.sin(i). You can swap these numbers to change whether the ellipse is longer horizontally or vertically. These numbers can also be changed to alter the length and width of your ellipse.