Scripting Book
Introduction
What is the Scripting Book?
The Scripting Book was created by Legokid135.
Basics
Output
The output is a window you can open in ROBLOX Studio by going to View -> Output. By default, the output window will run along the bottom of the ROBLOX Studio window.
Output displays many things such as bugs (errors) in your code, text you print (we will learn about print in the next subheader), etc etc etc.
Printing
In scripts, to print doesn't mean to print a document onto paper - it means to write text (e.g-in javascript you use document.write('text'), but we use print('text') in Lua.) into the output window. Insert a script into workspace by opening ROBLOX Studio and going to Insert -> Object -> Script -> OK.
Open up Explorer, press the '+' sign next to Workspace and find 'Script'. Double click on 'Script' and you will see there is already a line of code written for you. It should look something like this:
print 'Hello World'
Open up output window and press 'Run' found at the top right (by default) of ROBLOX Studio (should look like a Play Button.). Then the output window will have text in it. It should say
Running Script: Script
Hello World.
The reason for this is because you used the print function to print the text inside the quotes into the output window. If you changed Hello World to 'blah', your script would look like this,
print 'blah'
Then the output would display
Running Script: Script
blah
There are multiple ways of printing, such as followed:
- print 'Hi'
- print('Hi')
- print("Hi")
- print[[Hi]]
Let's Begin
These are the basics of scripting.
Your first script
ROBLOX's scripting language is their version of Lua. We call it RBX.lua (Short for ROBLOX Lua). It's not LUA, it's Lua. Lua is not an acronym and should not be written like one, or scripters will eat you.
In ROBLOX Studio, open up explorer (View -> Explorer) you see many different directory folders. It should look something like this:
If we wanted to locate a descendant (item inside) of 'Workspace', we would write a path script to it. All the services (folders you see in explorer) are descendants of 'game'. There are three ways of accessing workspace by using a script, these are
game.Workspace Workspace workspace
Let's say we have a WedgePart in Workspace and we wanted to delete it. We would locate the WedgePart and use the :Destroy() method to set it's parent to nil, in other words, deleting it.
game.Workspace.WedgePart:Destroy()
game.Workspace is the ascendant, or directory, of the instance named WedgePart. The :Destroy() method sets the instance's parent to nil, causing it to be deleted.
That code above does exactly what it says on the tin. It locates WedgePart, and it destroys the WedgePart.
Variables
First of all, what is a variable? A variable is something that holds data. You can have Numbers, Strings, Booleans and Objects.
You know what a number is, I hope. But what is a string? A string is something that holds words, letters... Generally any keyboard characters and can be found between speech marks, quotation marks and [[ and ]]'s. A boolean is something that contains two values, false and true. Object variables? What's an object variable? It's a variable that contains objects.
How does a variable 'think'? Well, here's a diagram of it:
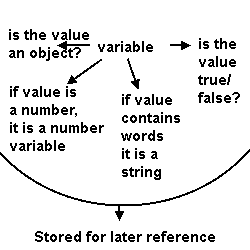
The diagram to the left shows the variable and what its job is. As an example, if the value of the variable was a number, it's a number value. If the value is either true or false, it's a boolean.
Well, how do you MAKE a variable? Let me assure you, making a variable is extremely easy. It's not like some other programming languages in which you have to define what type the variable is. Like in Java, you have to type:
Type Name = Value Boolean On = true
In Lua, we remove the type, so it gives us:
Name = Value
The 'Name' can be anything you want! Here's a couple of examples;
On = Value Hatred = Value Pizza = Value Friends = Value
What about the value? As we learned earlier, the value can be anything and the API can tell what type the variable is, so that saves us the extra work!
Here's a few examples;
On = false Hatred = true Pizza = true Friends = 1336
Cool! What else can we do with these 'variables'? Well, later on we're going to learn about the if statements. For now, we can use what we've learned to do our algebra homework! Oh, yes!
Wait a minute, I just remembered! I have some algebra homework. Oh no... Wait, with Lua, it's going to be FUN and EASY!! Here's what my homework is:
If the value of x is 5, what is the value of y if y is x + 3?
Well, I'm dumb. I don't know how to figure this out. It's too advanced for me. Fortunately, Lua is not dumb! So let's map this out.
'If the value of x is 5'. Hmm.. I know! I'll use a variables,
x = 5
'what is the value of y if y is x + 3?'. Does this mean I have to create a new variable for y? Well, in some cases yes, in other cases no. In this case we don't need to, but we will for future reference.
x = 5 y = 0
Now, we're going to do the arithmetic's.
x = 5 y = 0 y = x + 3
Now the value of y is no longer 0, it's x + 3, which is the same as saying 5 + 3, which equals 8. But we're not done yet! We have to get the computer to reply with the answer. So we're going to use the print function that prints the value of y, which is x + 3, which is 8.
x = 5 y = 0 y = x + 3 print(y)
Would output: 8. Yey! My algebra homework is complete. Let's think of easier ways of doing this:
x = 5 y = x + 3 print(y)
Well, it's one line smaller. Let's try and make it even smaller!
x = 5 print(x + 3)
... I'm still not happy. Can we slim this to one less line? I'd be happy to substitute the variable x!
print(5 + 3)
Hurrah! Now, we've covered the number variables. What about the others?
The second variable type we're going to learn about are Strings. Here's an example of one:
myString = "Hello World" print(myString)
The above example will print Hello World in the output window when the script is ran. Here's another example,
myString = "I just came from the world of zeros and ones!" print(myString)
Would print I just came from the world of zeros and ones!.
What about joining two variable strings together? We separate them by a comma:
myString = "Hello" mySecondString = "World" print(myString, mySecondString)
The above would result in 'Hello World'.
How about joining one string to another variable? Well, to do this we use two dots ( .. ) as found here:
myString = "World" print("Hello " .. myString)
Hmm.. What about two strings in between a variable?
myString = "Five" print("I have " .. myString .. " friends")
Would result in 'I have Five friends'.
More examples:
myNumber = 5 myString = "friends" myOtherString = "I have" print(myOtherString, myNumber, myOtherString)
Would result in: I have 5 friends
print("I need " .. 1 .. " chocolate bar in " .. 5 .. " days!") print("I need 1 chocolate bar in 5 days!") print("I need", 1, "chocolate bar in", 5, "days!")
All three of the above examples will output: 'I need 1 chocolate bar in 5 days!'.
Cool! How about Booleans? First of all, what are booleans? Well, booleans consist of only two values, true and false. Later, when we use if statements, we will be able to check if a boolean's value is true, if it is, change its value to false and move on.
What use do booleans serve us? Creating a "debounce" mechanism is one example.
How do we set up one? It's quite easy, really;
myBoolean = false
The value of the myBoolean variable is set to false. Let's use what we've learned to create a small snip of code.
myBoolean = false print(myBoolean)
The above example will output 'false'.
myBoolean = false myString = "True or" print(myString, myBoolean .. "?")
The above example prints 'True or false?'.
More examples:
print(false .. "!")
The above prints 'false!'.
print(true .. " or " .. false .. "?")
The above prints 'true or false?'.
print(true, "or", false , "?")The above prints 'true or false?'.
Mathematics in RBX.lua?
You can use a variable to hold mathematics, pretty much like algebra! Then you can print the variable into the output window and there's your math homework completed.
e = 45 b = e + 5 print(b)
Would print 50 in output, because b's value is e's value, which is 45, plus 5. So b's value is 5 more than e's value, making b's value 50. So when we print b's value in output, it will print 50.
Loops
What is a loop? A loop is something that does the same thing over and over, some loops go on forever, some loops stop when a condition returns differently.
There are three kinds of loops:
while CONDITION do end
for i = NUMBER, INCREMENT do end
repeat wait() until CONDITION
The first example is ofternly used as an infinate loop as such shown below:
while true do wait() end
The second example repeats the same thing over and over for a given amount of time, example, if we wanted to print 'Hello World' in output 5 times, we would do this:
for i = 1, 5 do print 'Hello World' end Would result in > Hello World > Hello World > Hello World > Hello World > Hello World
And the last example repeats the same thing over and over until a condition is changed. For example,
repeat wait() game.Workspace.Part.Position = game.Workspace.Part.Position + Vector3.new(1, 0, 0) until game.Workspace.Part.Position.X >= Vector3.new(10, 0, 0)
You can do the the second example and the third example as infinate loops by doing this:
for i = 1, math.huge do end
repeat wait() until false
Instance
Instance is a table that contains all the objects you can find when you open up ROBLOX Studio and go to Insert -> Object. Instance creates a object that you have selected from the object table and parents it in the given directory (or nil if not given a directory).
We want to create a variable. It can be named anything you want. I am calling my variable 'WP' - short for WedgePart, because I want to create a WedgePart.
WP =
Next we need to use Instance, but, since we are creating an object from the table we need to put '.new' after Instance and an open parenthesis (braket).
WP = Instance.new(
Now we need to tell the script what object we wish to pull out of the object table. Which my choice was WedgePart, but we need to tell the script that this is a String so we're going to put speechmarks around WedgePart.
WP = Instance.new("WedgePart"
That's good so far, now we're going to parent it in Workspace. So we're going to put a comma after the string. The comma seperates the two arguments, then we're going to put game.Workspace and then a close braket.
WP = Instance.new("WedgePart", game.Workspace)
The example above creates a WedgePart and puts it in Workspace.
WedgePart's Properties
Every object has properties. Here's a list of a WedgePart's properties:
BrickColor
Material
Reflectance
Transparency
ClassName
Name
Parent
Position
RotVelocity
Velocity
Anchored
Archivable
CanCollide
Locked
ResizeIncrement
ResizableFaces
Elasticity
Friction
Size
formFactor
BackParamA
BackParamB
BackSurfaceInput
FrontParamA
FrontParamB
FrontSurfaceInput
LeftParamA
LeftParamB
LeftSurfaceInput
RightParamA
RightParamB
RightSurfaceInput
TopParamA
TopParamB
TopSurfaceInput
BackSurface
BottomSurface
FrontSurface
LeftSurface
RightSurface
TopSurface
Accessing These Properties
To access these properties, we need to locate the WedgePart,
game.Workspace.WedgePart
Next we need to add a period. This period will tell the script that we're going to access one of it's descendents or properties. In this case, property.
BrickColor Property
First, we're going to edit the BrickColor property. So after the period we add BrickColor.
game.Workspace.WedgePart.BrickColor
Next, we're going to add an equals sign, to tell the script that we're going to edit that property. Then after the equals we need to add BrickColor.new to tell the script we're changing the value of that property and then an open braket.
game.Workspace.WedgePart.BrickColor = BrickColor.new(
There are many different colors to choose from. I am changing the WedgePart's color to Bright blue because I like that color. So we need to put speechmarks around the value to tell the script that we're going to insert a script, then a close braket to tell the script where the function stops.
game.Workspace.WedgePart.BrickColor = BrickColor.new("Bright blue")
Material Property
The material property contains 9 Enum values.
Plastic | Wood | Slate | Concrete | CorrodedMetal | DiamondPlate | Foil | Grass | Ice |
0 | 1 | 2 | 3 | 4 | 5 | 6 | 7 | 8 |
They can be edited by using the Material property.
game.Workspace.WedgePart.Material = ENUM_VALUE
Where it says 'ENUM_VALUE' we can change that to one of the values above. For example, if ENUM_VALUE was 0, the material of WedgePart would be Plastic, if it was 6 the material would be Foil.
Reflectance
The reflectance property goes from 0 to 1. 0 being not shiny atall and 1 being extremely shiny. 0.5 being in the middle.
game.Workspace.WedgePart.Reflectance = 0.5
Transparency
The transparency property goes from 0 to 1. 0 being opaque and 1 being invisible. 0.5 being transparent.
game.Workspace.WedgePart.Transparency = 0.5
ClassName
The ClassName property can not be changed. This property determinds what this object is.
Name
The name property is set to the kind of object by default. If we change this property, whenever we next use a path to it, we have to use its new name. We need to put speechmarks around its value to tell the script that the name is a string
game.Workspace.WedgePart.Name = "Hai"
Parent
The parent property determinds which directory the object is a descendant of. If we changed it to something other than Workspace, the object will not be visible and all scripts descending from that object will be disabled.
game.Workspace.WedgePart.Parent = game.Workspace
Position
The position property contains three values, since the game is 3D (or 2.5D however you want to say it), the values are in axes, X axis, Y axis and Z axis.
To change this property we need to use Vector3.new and an open braket to tell the script we're changing the WedgePart's position.
game.Workspace.WedgePart.Position = Vector3.new(0, 0, 0)
The first 0 is the X axis, the second 0 is the Y axis and the last 0 is the Z axis.
Anchored
The anchored property determinds whether or not the object can fall. It's a boolean, meaning that it can only have two values, true or false. If it's value is true, the object cannot fall. If the value is false, the object can fall
game.Workspace.WedgePart.Anchored = true
Archivable
The archivable property is also a boolean. If it's value is true, the object will not be visible when the place is published and it cannot be cloned.
game.Workspace.WedgePart.Archivable = false
CanCollide
The cancollide property determinds whether or not you can walk through the object. This property is also a boolean. If it's value is true, you cannot walk through it. If it's false then you can walk through it.
game.Workspace.WedgePart.CanCollide = false
Locked
The locked property determinds whether or not you can move the object with the mouse. If it's value is true, you cannot drag the object with the mouse in/out of ROBLOX Studio, if it's value is false, you can drag the object with the mouse.
game.Workspace.WedgePart.Locked = true
Size
The size property is like the position property, only it resizes the object instead of re-positioning it the reason it is simillar to the position property is because it also uses the 3 axes, X,Y and Z. We also still use Vector3.new(x,y,z) to edit it's values.
game.Workspace.WedgePart.Size = Vector3.new(5, 5, 5)
FormFactor
The FormFactor property is one I always mispell as FormFactory, so I have to be careful here. The FormFactor property is used to change the form of the object.
This also uses Enum values.
Symmetric | Brick | Plate | Custom |
0 | 1 | 2 | 3 |
game.Workspace.WedgePart.FormFactor = 3
Mathematical Signs
Table of mathematical signs that will help us later in the book:
To the power of | Percent | Divide | Multiply | Plus | Subtact |
^ | % | / | * | + | - |