Vector2
Vector2
A Vector2 has two values - an X ordinate, and a Y ordinate. They're kind of like those coordinates you use in school on graphs. I'm sure you've seen something like
(1, 5)
somewhere before. This means that on a graph you go to the right 1, and then up 5. That's because a coordinate uses an X and a Y value. It looks like this
(x, y)
That's really all there is to it. Usually, the use of Vector2s are in doing simple calculations that UDim2s do not have built in. When people talk about the values inside a Vector2,
- X is horizontal, or width
- Y is vertical, or height
Constructors
Constructor | Description |
---|---|
Vector2.new(x, y) | Creates a new Vector2 using ordinates x, and y. |
Properties
All of these properties are Read Only (you can't just set them Vector2.x = 5, it doesn't work) but you can create new vectors with such changes, or apply an operation, seen in the next section.
Property | Type | Description |
---|---|---|
Vector2.x | number | The x-coordinate |
Vector2.y | number | The y-coordinate |
Vector2.unit | Vector2 | A normalized copy of the vector |
Vector2.magnitude | number | The length of the vector |
Magnitude property
The magnitude property is simply the length of the Vector2, which can be found by using the pythagorean theorem. If you plug-in the difference of the Vector2's x coordinates as a and the difference of the Vector2's y coordinates b, and then solve for c, you will find the length of the Vector2. If there is only one coordinate, the second coordinate will be
.
Operators
Operator | Description |
---|---|
Vector2 + Vector2 | returns Vector2 translated (slid) by Vector2 |
Vector2 - Vector2 | returns Vector2 translated (slid) by -Vector2 (also gives relative position of 1 to the other) |
number * Vector2 | returns Vector2 with each component multiplied by number |
Vector2 * number | returns Vector2 with each component multiplied by number |
number / Vector2 | returns Vector2 with number divided by each component |
Vector2 / number | returns Vector2 with each component divided by number |
Vector2 * Vector2 | returns Vector2 with each component multiplied by corresponding component |
Vector2 / Vector2 | returns Vector2 with each component divided by corresponding component |
Examples
In the following diagram, there are two line segments. These two line segments can be represented in Roblox with four Vector2 values.
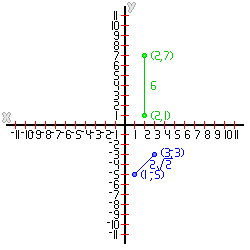
Let's say we have line A:
Now, if we were to print the length (or magnitude) of each line segment, it would be the number displayed to the right of it: